What is React Query?
React Query is a powerful library for managing server-state in React applications. It’s like a helpful assistant that takes care of fetching, caching, and updating your data, making sure everything runs smoothly. Think of it as a smart manager that handles all the data requests and ensures your app stays up-to-date without you having to worry about the details.
What are the advantages?
Using React Query offers a range of benefits that can significantly improve your development experience and the performance of your application. Here are some key advantages:
- Simplified Data Fetching: React Query handles the process of fetching data from your server, so you don’t have to write repetitive code. This saves time and reduces the chance of errors.
- Automatic Caching: It automatically caches the fetched data, making your app faster and more efficient. No need to fetch the same data repeatedly!
- Background Updates: React Query can refetch data in the background, ensuring your app always displays the most current information without disrupting the user experience.
- Error Handling: It provides built-in tools for managing errors, making it easier to display meaningful messages to users when something goes wrong.
- Optimistic Updates: Your app can feel more responsive by immediately showing changes while the actual update is being processed in the background.
Caching fetches and refetching in background
One of the standout features of React Query is its smart caching system. When data is fetched, React Query stores it in a cache. If your app needs the same data again, it will use the cached version, speeding up the process and reducing unnecessary server requests.
But what if the data changes? React Query can refetch the data in the background, ensuring your app always has the latest information. This means users get a fast, smooth experience without waiting for data to load each time they navigate through the app.
How invalidation works
Invalidation is like hitting the refresh button on specific data. When some data in your app changes, React Query knows it needs to fetch fresh data. This process is called invalidation.
For example, if a user updates their profile, React Query will mark the profile data as “stale.” It then refetches the updated profile information, ensuring the app displays the most recent data. This way, users always see the latest information without having to manually refresh the page.
Better UX
React Query significantly enhances user experience (UX) by making data handling seamless and efficient. Here’s how:
- Faster Load Times: Cached data means quicker load times, reducing the waiting period for users.
- Real-Time Updates: Background refetching ensures users always see the latest data without manual refreshes.
- Responsive Interfaces: With optimistic updates, users see immediate changes, making the app feel more responsive.
- Error Transparency: Better error handling provides clear messages to users, keeping them informed without confusion.
Overall, React Query contributes to a smoother, more responsive, and reliable application, which keeps users happy and engaged.
Fetching with React Query vs standard fetching
Standard
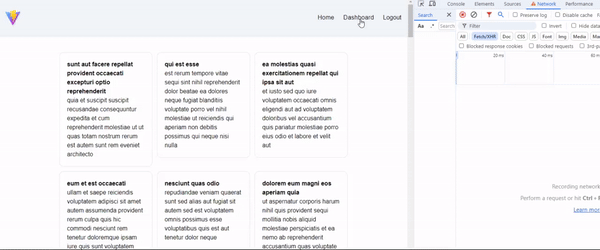
React Query
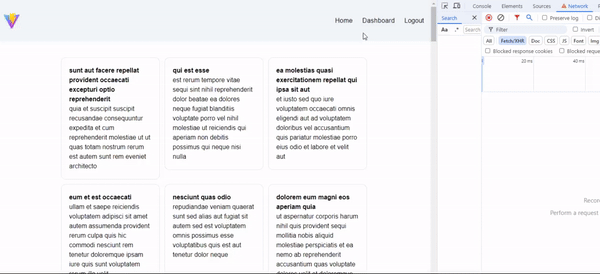
As you can see data fetched with React Query is already cached when navigating between pages it boosts performance and user experience really well!
Also in the background react query makes additional request to compare datas to check if there’s newer version out of the box!
Example implementation
It is easy to get started and maintain the codebase. Below you can find how simple it is.
1. Basic Setup
First, let’s install React Query:
npm install @tanstack/react-query
Next, wrap your application with the QueryClientProvider
to enable React Query in your project:
// index.js or App.js
import React from 'react';
import ReactDOM from 'react-dom';
import { QueryClient, QueryClientProvider } from '@tanstack/react-query';
import App from './App';
const queryClient = new QueryClient();
ReactDOM.render(
<QueryClientProvider client={queryClient}>
<App />
</QueryClientProvider>,
document.getElementById('root')
);
2. Simplified Data Fetching
Here’s a basic example of fetching data with React Query:
// App.js
import React from 'react';
import { useQuery } from '@tanstack/react-query';
const fetchPosts = async () => {
const res = await fetch('https://jsonplaceholder.typicode.com/posts');
return res.json();
};
const Posts = () => {
const { data, error, isLoading } = useQuery({
queryFn: () => fetchPosts(),
queryKey: ["posts"],
})
if (isLoading) return <div>Loading...</div>;
if (error) return <div>Error: {error.message}</div>;
return (
<div>
{data.map(post => (
<div key={post.id}>
<h3>{post.title}</h3>
<p>{post.body}</p>
</div>
))}
</div>
);
};
export default Posts;
Conclusion
React Query is a game-changer for managing data in React applications. By simplifying data fetching, caching results, and handling background updates, it ensures your app is always fast, reliable, and up-to-date. The automatic invalidation keeps the data fresh, while the improved user experience makes your app more enjoyable to use. Embracing React Query can streamline your development process and lead to happier users, making it a valuable addition to any React project.