In today’s Flutter world, state management is a crucial aspect of every application. There are several approaches to state management, but two of them—BLoC (Business Logic Component) and Riverpod—have gained popularity due to their effectiveness and flexibility. In this post, we’ll compare these two approaches to help you understand which one might be best for your project.
BloC is one of the most well-known state management patterns in Flutter. The principle behind BLoC is to separate business logic from the user interface, leading to greater modularity and easier testing.
On the other hand, we have Riverpod, which is a modern state management solution. It addresses many issues found in other state management libraries by offering a more flexible and secure API.
The question of whether one is better than the other depends on various factors, such as the complexity of your application, your experience as a programmer, and your personal preferences. In this post, we will closely examine both approaches to help you make an informed decision.
bloc
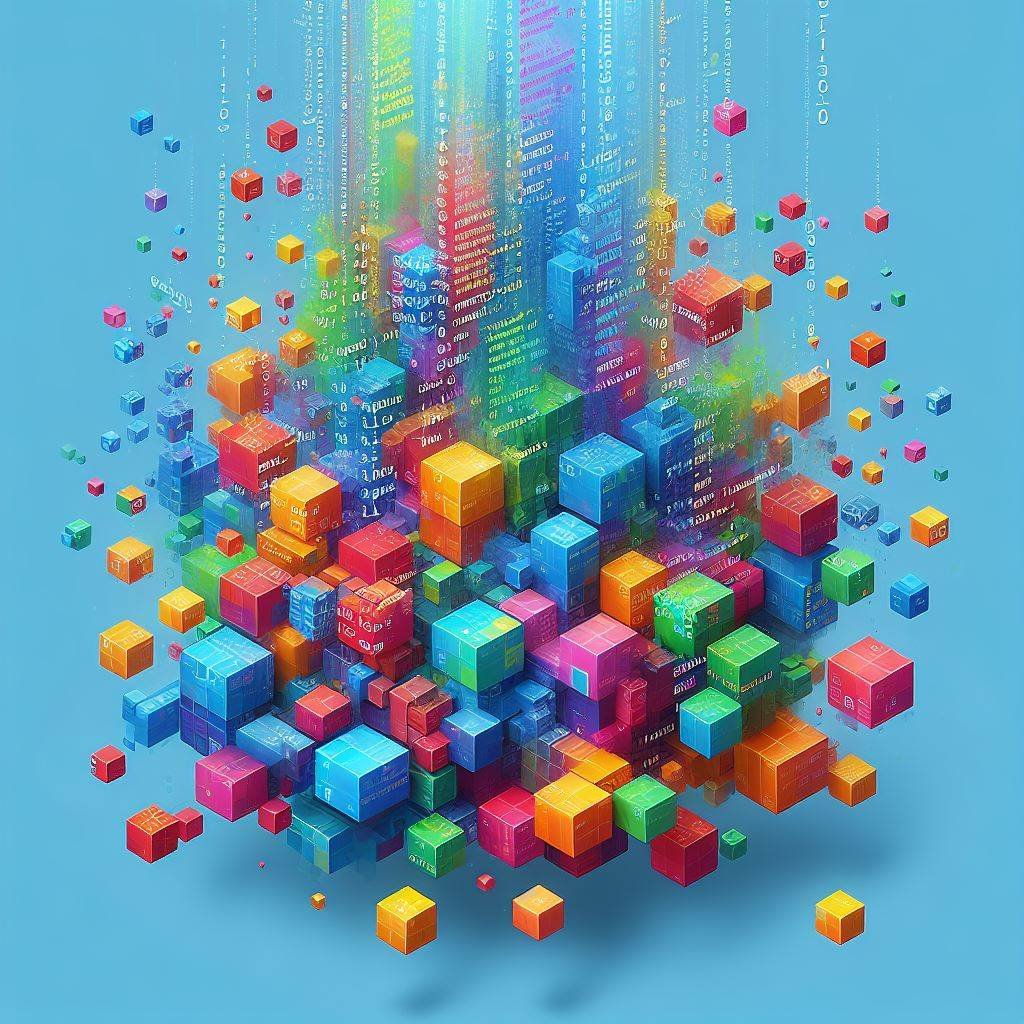
Bloc is a design pattern that enables programmers to efficiently and conveniently manage state in Flutter applications. The key feature of BLoC is the separation of business logic from the user interface, allowing for flexible data management.
Bloc has the following advantages:
- Architecture: BLoC follows an architectural pattern that helps separate business logic from the presentation layer (view). This makes the code more modular and easier to test.
- Events and States: In BLoC, we define events (actions) that represent user interactions (e.g., button clicks). Based on these events, BLoC generates states that describe the current application state (e.g., loaded data, errors).
- Testability: BLoC is test-friendly. We can verify whether our logic works correctly by simulating different events and checking expected states.
However, there are some drawbacks:
- Complex Code: Implementing BLoC can be time-consuming and lead to extensive code.
- Boilerplate: Creating events, states, and event handling can be cumbersome.
Below, you can observe the code written in Bloc in the main.dart
file for the famous CounterApp example. In the MyApp
class, only a BlocProvider
has been introduced. Then, in MyHomePage
, a BlocBuilder
has been declared to read the given counter, and in the onPressed
function, a method for incrementing the counter has been introduced.
main.dart
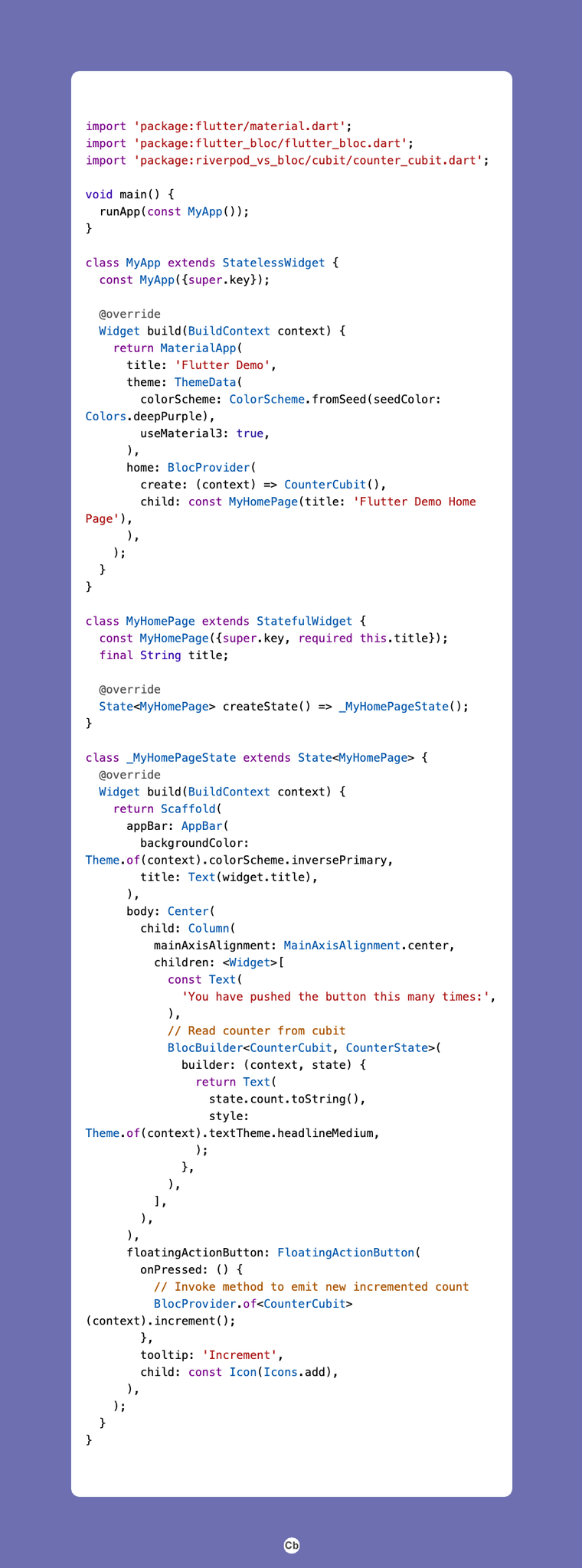
Okay, but to input data, we need to create two separate classes: Cubit
and State
. For this purpose, I also utilized the freezed
package to generate the State
(although it’s not necessary). In the State
class, I declared a method for incrementing and a count
field.
counter_state.dart
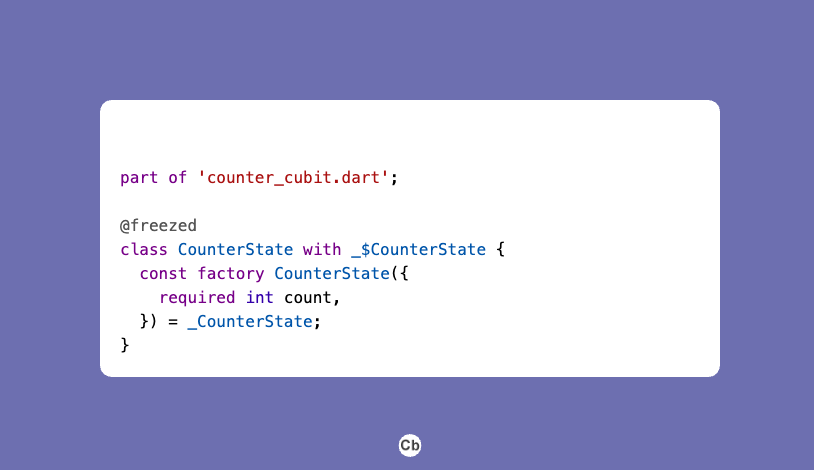
counter_cubit.dart
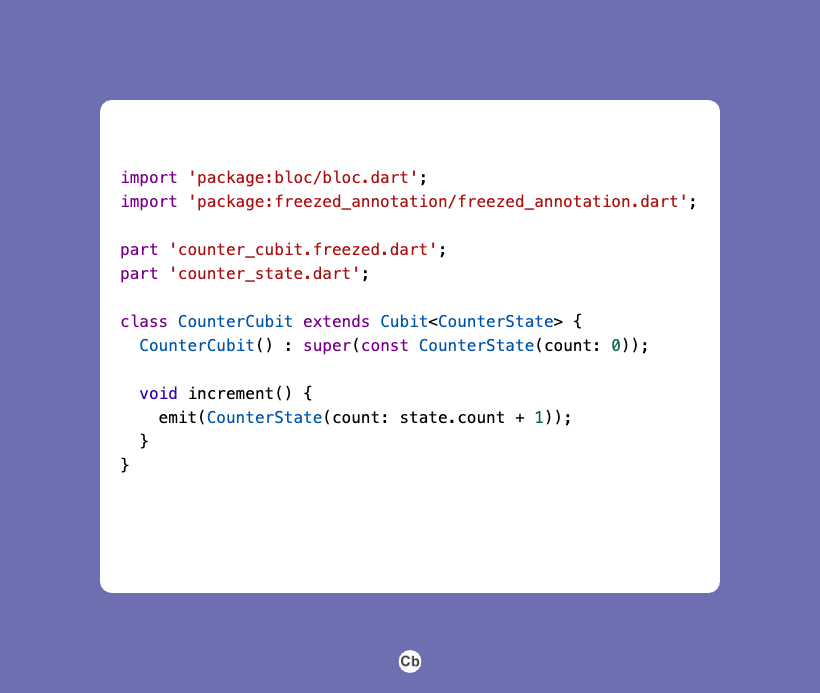
And That’s minimum for BLoC pattern (cubit).
riverpod
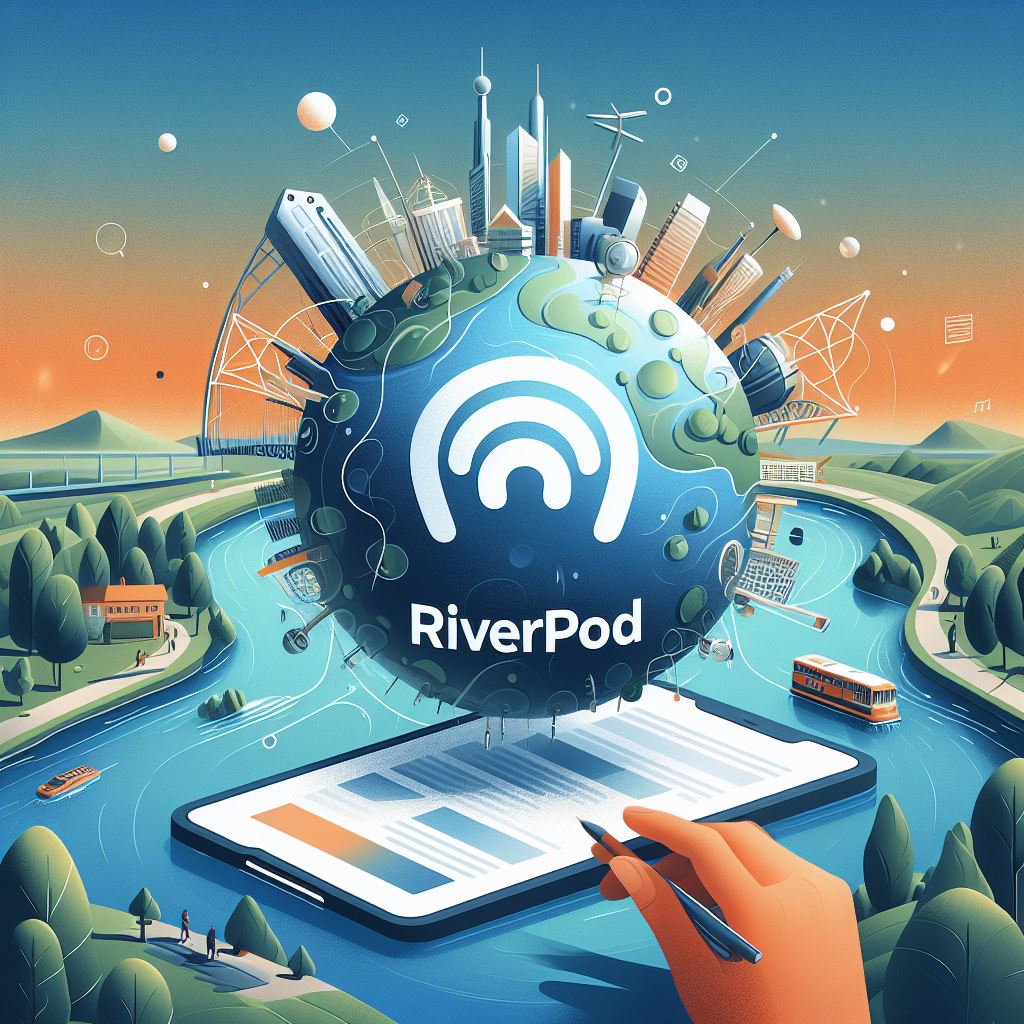
Riverpod is a reactive framework for state management in Flutter/Dart that has gained popularity due to its unique features. Let’s explore its advantages:
- Simplicity: Riverpod serves as a more minimalist alternative to BLoC, making it easier to understand and use.
- Dependency Injection: Riverpod provides built-in support for dependency injection, simplifying state management.
- Testability: Similar to BLoC, Riverpod is test-friendly.
And the disadvantages are less popularity and the lack of enforced architecture separating presentation from logic.
Below is the code created based on the same CounterApp. In MyApp, we can see that, similar to Bloc, we register our ProviderScope to have access to all providers we create. Then in MyHomePage, counterStateProvider is declared, handling our counter exchange. Next, we have two options: either transform State<MyHomePage> into a ConsumerWidget and load it with build ref, or use a local Consumer widget.For this example, I chose the second option to observe the counter state in the Text widget and for the onPressed FloatingButton.
main.dart
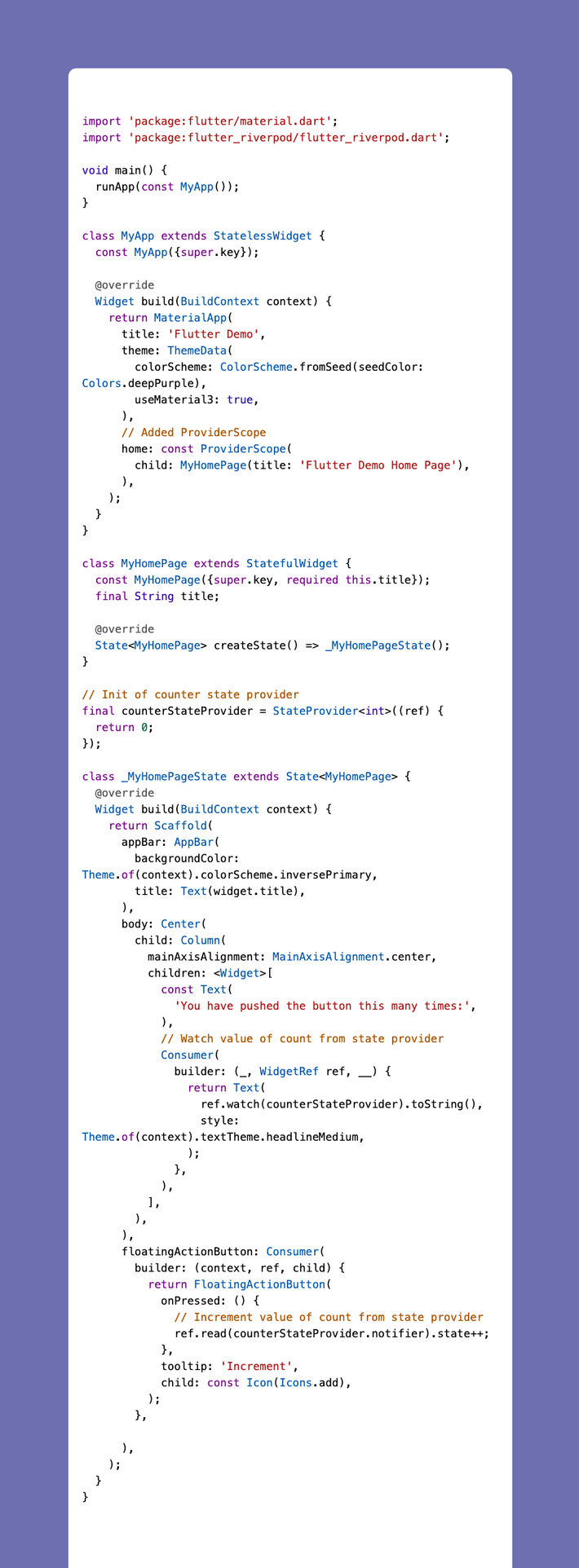
summary
Both Riverpod and Bloc are powerful tools for managing state in Flutter applications. The choice between them depends on the developer’s preferences. If you have prior experience with state management in React, there’s a good chance that you’ll appreciate Riverpod with its hooks support.
Riverpod is also a package that requires minimal configuration (e.g., no need to create a separate bloc, states, or events classes). Therefore, if you’re working on a small project where application architecture isn’t the primary concern, Riverpod might be an ideal choice. On the other hand, Bloc enforces separation of presentation code from business logic, which aligns well with more advanced architectures (though Clean Architecture can also be applied with Riverpod, depending on the developer’s preference).
It’s worth noting that both tools have their merits and use cases, so the choice ultimately depends on the project’s specifics and individual preferences. 🚀