GitHub Copilot is an artificial intelligence-based tool that assists in code generation. It can aid in creating unit tests and widgets, but the process is not entirely automatic and requires the user to have a certain level of knowledge and skills.
Unit tests, widget tests, and integration tests are the three levels of testing in Flutter. Each of them has its specific use and purpose.
Unit tests: Unit tests are the simplest level of testing. They are used to test individual functions, methods, or classes. The goal is to verify that a specific unit of code works correctly in isolation. In Flutter, unit tests are typically written using the test package.
Widget tests: Widget tests are used to test individual widgets in isolation. The goal is to check whether widgets correctly respond to various inputs and state changes. Widget tests are more complex than unit tests as they need to simulate user interactions, such as clicks or swipes. In Flutter, widget tests are written using the flutter_test package.
Integration tests: Integration tests are used to test the entire application as one system. The goal is to verify that different parts of the application work together correctly. Integration tests are the most complex but also the closest to real user usage of the application. In Flutter, integration tests are written using the flutter_driver package.
Remember that each testing level has its place, and it is important to use all of them to ensure the highest code quality.
An example of unit test
Copilot assists in writing unit tests for functions. Let’s consider the function
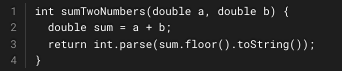
We create a new file in the test folder named sum_two_numbers_test.dart, where the suffix (_test.dart) is required by Flutter. Click (command + i) on the newly opened file and enter the command:Write unit tests for the function [paste this function] considering additional various use cases for this function.
After generating the test, the file looks like this:
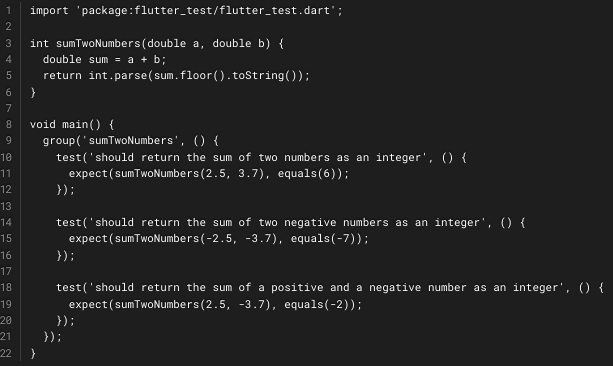
Copilot can generate tests with cases we might not even have thought of.
An example of widget test
The additional tests that Copilot can generate are widget tests. In addition to function tests, we can generate tests for specific widgets. These tests help locate and verify the behavior of a widget subtree.
Let’s consider the widget.
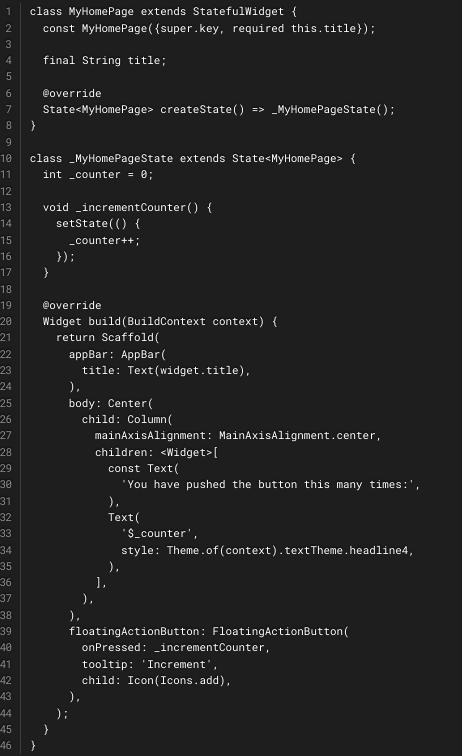
We can generate tests for the mentioned widget using Copilot. To do this, we create a new file in the test folder and name it, for example, myhome_page_test.dart. We open the command window (command + i) and type:
"Create a widget test
[paste widget code]
checking the following:
a) whether the initial value of the counter variable is 0
b) whether clicking the button containing the Icons.add icon increases the counter value by 1"
The result
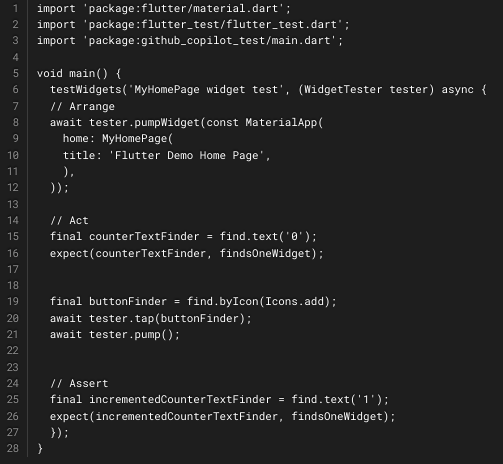
We run the test and check if it passed successfully. In this case, the test was conducted without errors.
integration tests
These are more complex tests that allow checking the operation of the entire application, including navigation between screens, etc. Copilot can assist in generating this type of test, but it may be challenging for it to generate the entire test.
SUMMARY
The article discusses GitHub Copilot, an AI-based code generation tool, emphasizing its assistance in creating tests to ensure high code quality, albeit with some user involvement.