In today’s world, as mobile applications become increasingly complex, developers are looking for new tools and technologies that will help them meet growing demands. One such tool that has gained popularity in the Flutter world is RxDart.
RxDart is an implementation of ReactiveX (Rx) for the Dart language, which is the foundation of Flutter. With its powerful operators and streams, RxDart opens up new possibilities for Flutter developers, enabling them to create more reactive and dynamic applications.
While Dart already has a good stream API, RxDart adds functionality based on the Reactive Extensions specification. It’s important to note that RxDart doesn’t replace Dart Streams but provides additional stream classes, operators (methods extending the Stream class), and Subjects.
In this article, we will take a closer look at RxDart, understand how it works, and how it can contribute to building better applications in Flutter. We will also analyze a few examples that will show how to effectively use RxDart in practice.
what’s mean reactive?
Reactive Programming is a programming paradigm that focuses on handling data streams and events. It’s a declarative approach that is based on the idea of asynchronous event processing and data streams.
In this paradigm, it’s possible to express static (e.g., arrays) or dynamic (e.g., event emitters) data streams with ease, and to communicate that there is an inferred dependency in the associated execution model, which facilitates automatic propagation of the changed data flow.
Currently, there are many reactive programming frameworks. The first reactive programming library was RxJava, which was introduced in 2013. Today, the reactive programming paradigm is also applied in cloud applications. Cloud-based services are often implemented as a set of microservices. These are small components that are loosely coupled and communicate with each other via asynchronous message passing.
what is it
RxDart extends the capabilities of Dart streams and stream controllers. Dart has a very decent stream API; instead of trying to provide an alternative to this API, RxDart adds functionality from the reactive extensions specification.
RxDart does not provide its Observable class as a substitute for Dart streams. (in older version it has own classes). Instead, it offers several additional stream classes, operators (extension methods for the Stream class), and Subjects.
practice
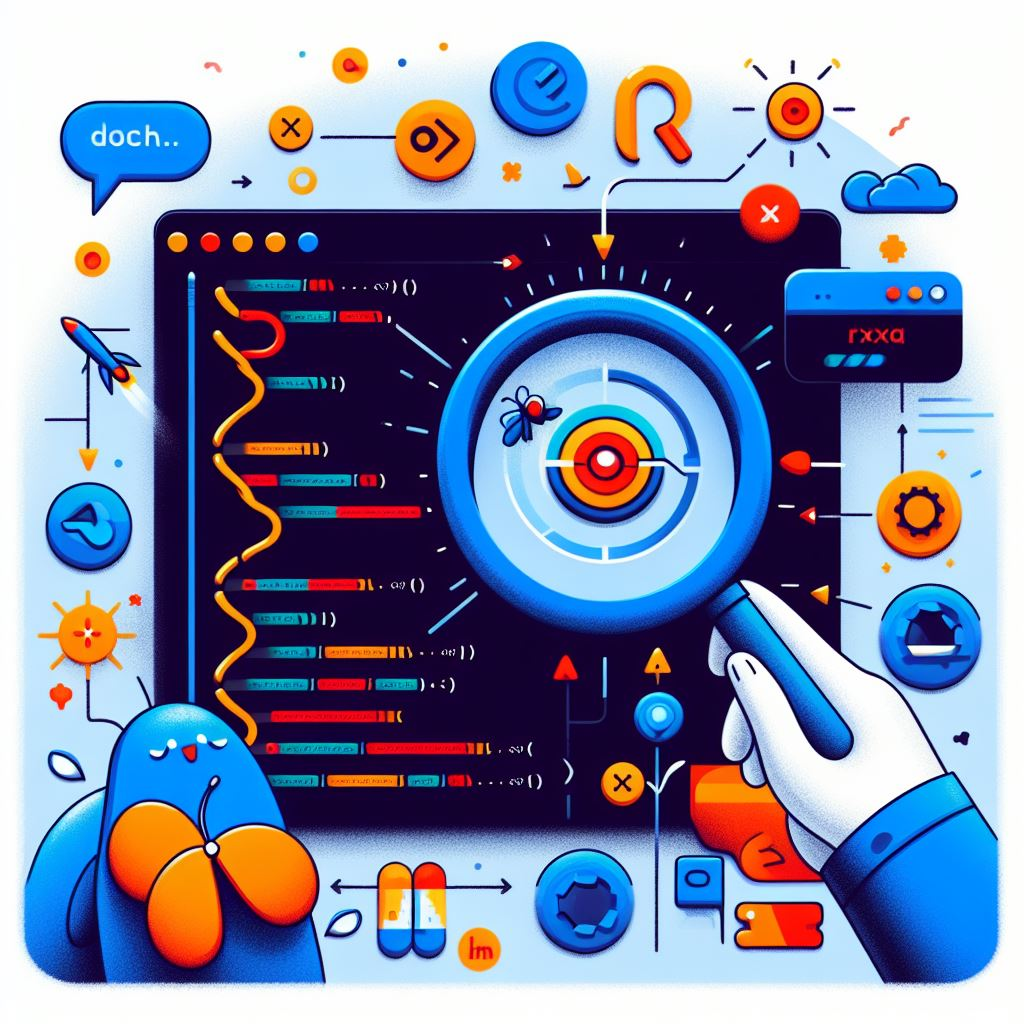
For example, assume we have a text field where the user can enter a search query. We want to send a query to the server only when the user stops typing for at least 500 milliseconds. We can do this using the debounceTime
operator provided by RxDart.
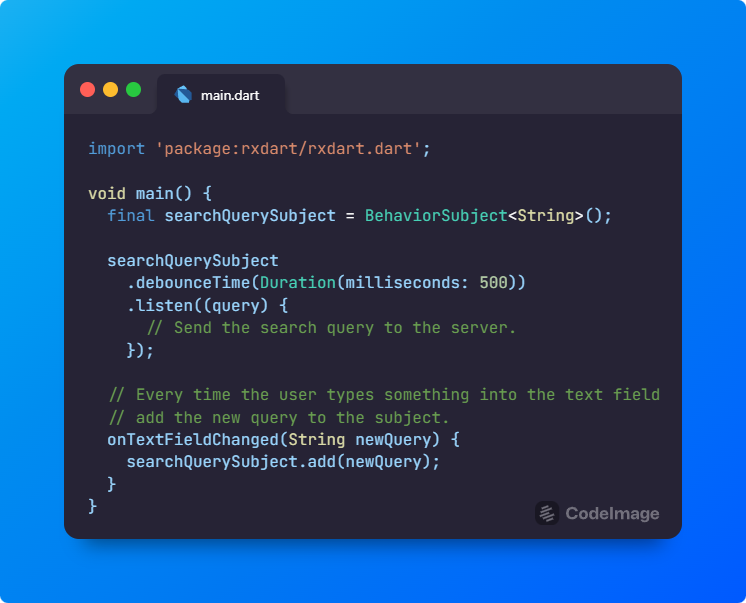
In this example, searchQuerySubject
is a Subject
that emits new search queries. Each time the user types something into the text field, the new query is added to searchQuerySubject
.
The debounceTime
operator waits 500 milliseconds after each new event. If no new event occurs during this time, it emits the last event. This means that the query to the server is sent only when the user stops typing for at least 500 milliseconds. This is very useful to avoid sending queries to the server too frequently.
Here are descriptions of several RxDart methods that are useful in reactive programming:
buffer
: Thebuffer
method allows you to collect elements from a stream and group them based on a specified count or condition. For example, you can usebuffer
to collect elements every 5 seconds or after a certain number of elements.delay
: Thedelay
method postpones the emission of elements from a stream by a specified duration. You can use this to introduce delays in processing or synchronize streams.endWith
: TheendWith
method adds specific elements to the end of a stream. For instance, you can append an element after the stream completes.exhaustMap
: TheexhaustMap
method ignores new events if a previous event is still being processed. It’s useful when you want to avoid concurrent processing of multiple events.groupBy
: ThegroupBy
method groups stream elements based on a specified key. You can use this to divide a stream into smaller groups.max
: Themax
method returns the maximum element from a stream based on a specified criterion (e.g., numeric value).sample
: Thesample
method emits the most recent element from a stream at regular time intervals. It’s handy for monitoring stream state periodically.scan
: Thescan
method accumulates values from a stream using a specified function. You can use it to calculate sums, averages, etc.window
: Thewindow
method creates a new stream containing a specified number of elements or elements within specific time windows.
And more methods and examples find in documentation of RxDart.
Popularity of RxDart in 2024
On GitHub, RxDart continues to attract significant interest. It currently has over 3,300 stars and is actively maintained by the community (freshes commits and merges). Valuable insights can be gained by analyzing star counts, pull requests, and repository activity.
Comparing RxDart with Dart Streams:
- RxDart offers more advanced operators and stream classes, making it easier to work with asynchronous data.
- Dart Streams is often used for simpler cases, while RxDart shines in more complex scenarios.
Regarding updates, RxDart is actively developed. The latest version, 0.28.0-dev.0, introduced new features like ValueStream and ReplayStream, along with documentation improvements and examples. Keeping an eye on the repository ensures you stay up-to-date with the latest releases.
In summary, RxDart remains popular in programming in 2024, and its development is actively supported by the community. If you’re working with asynchronous data in Dart, exploring this library is worthwhile.